题干
Given n points in plane, where , no two points coincide and no three points are collinear. Find two intersecting lines satisfying that no given points lie in the two lines and that for each of the four divided areas, there are exactly given points. If multiple solutions exist, print any one of them. If no solution, print “-1” in one line.
Input
The first line contains one positive integer , denoting the number of test cases. For each test case:
The first line contains one integer , denoting the number of given points.
Following n lines each contains two integers , denoting one given point .
It is guaranteed that , that no two points coincide and that no three points are collinear.
Output
For each test case:
If no solution, print “-1” in one line. Else print two lines each containing four integers with absolute value not exceeding , denoting one line passing simultaneously.
给定平面上的个点,其中,没有两个点重合,没有三个点共线。找出两条相交的直线,满足两条直线上没有给定的点,并且对于四个划分的区域中的每一个,都有给定的点。如果存在多个解决方案,则打印其中任何一个。如果没有解决方案,在一行中打印“-1”。
Input
第一行包含一个正整数,表示测试用例的数量。对于每个测试用例:
第一行包含一个整数,表示给定点的数量。
下面的n行每行包含两个整数,表示一个给定的点。
可以保证,没有两个点重合,没有三个点共线。
Output
对于每个测试用例:
如果没有解决方案,在一行中打印“-1”。否则打印两行,每行包含四个整数,绝对值不超过,表示一行同时传递。
测试案例
Input
1 | 2 |
Output
1 | 0 1 0 -1 |
题意与思路
给定 个点,其中 是 的倍数,任意两点不重合、任意三点不共线,要求在平面上找到两条相交的直线,这两条直线将平面划分为4个区域,每个区域恰好都有 个点。
对于某一条直线,通过 两点确定一条直线 的原则来定义,即给出两个点的坐标,来表征这一条直线。
我们考虑 先找一条直线将所有点平均分为两部分,再找第二条条直线将这两部分各自平分为两部分。
先找第一条直线
两条直线不好找,一条直线就没那么难了:
- 当位于中间的两个点 纵坐标不同 时,将它们的 纵坐标分别增加和减少一个足够大的值 ,得到第一条平分线
- 当位于中间的两个点 纵坐标相同 时,将它们的 横坐标分别增加和减少一个偏移量 ,再执行上一条选项,得到第一条平分线
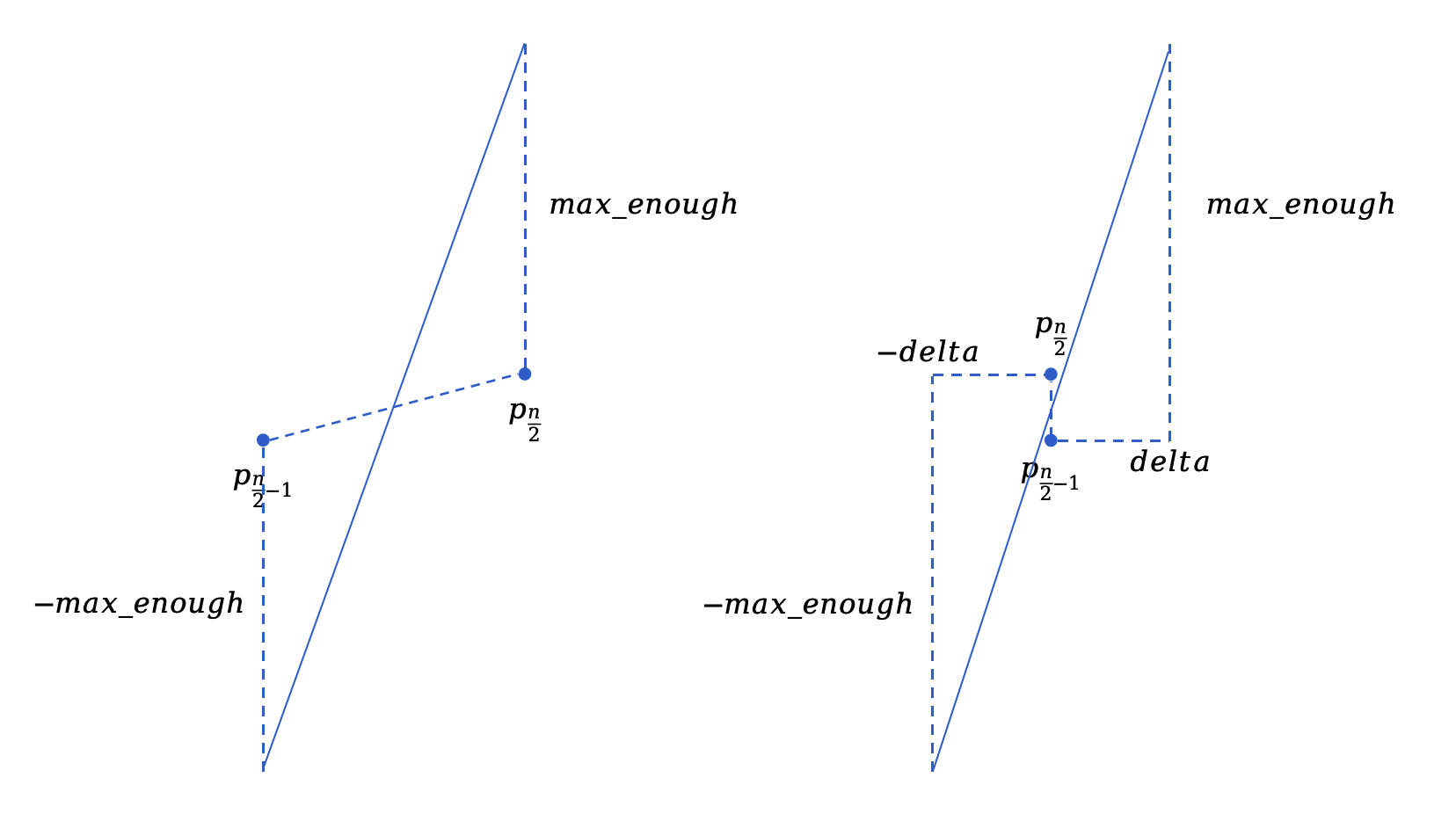
按照这样办法找到的直线 位于中间的两点之间,只要 足够大,这条直线将会无限接近于 垂直 x 轴,因此它 不会经过其他点。
至此,我们找到了第一条平分线。
ps. 有关 的取值,我采用了常量定义的形式尝试,因为本题要求的输出范围为 ,因此从 开始尝试,这一取值对本题的评测案例来说满足题意。
再找第二条直线
找到一条直线 后,还需要找到一条与它相交的直线 ,我们采用下面的思路来 二分 地查找:
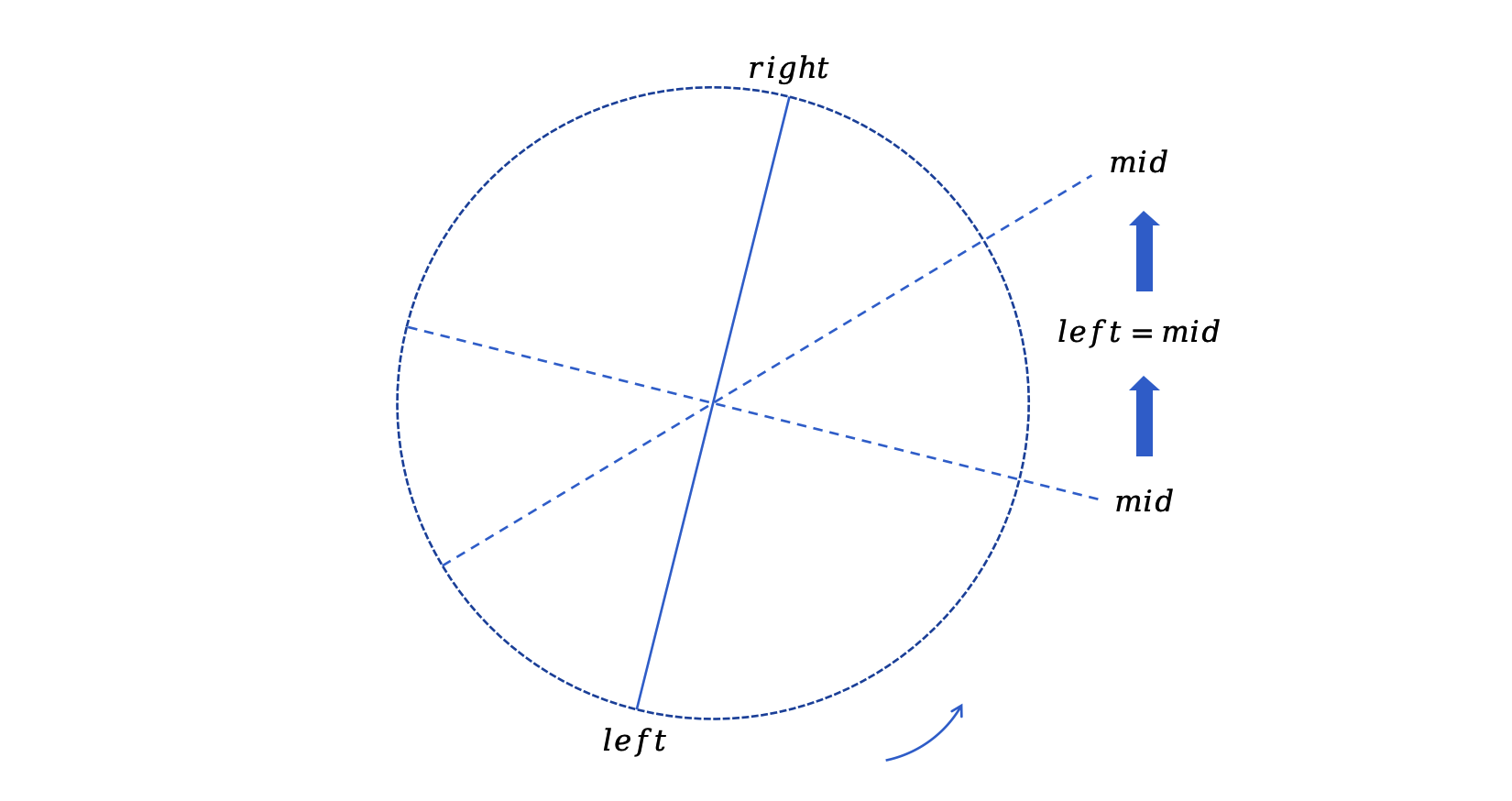
如上图所示,我们 二分查找第二条直线的斜率,对于任意一个确定的斜率(如下图粗虚线所示),我们将 个点按照这一斜率投影到直线 上:
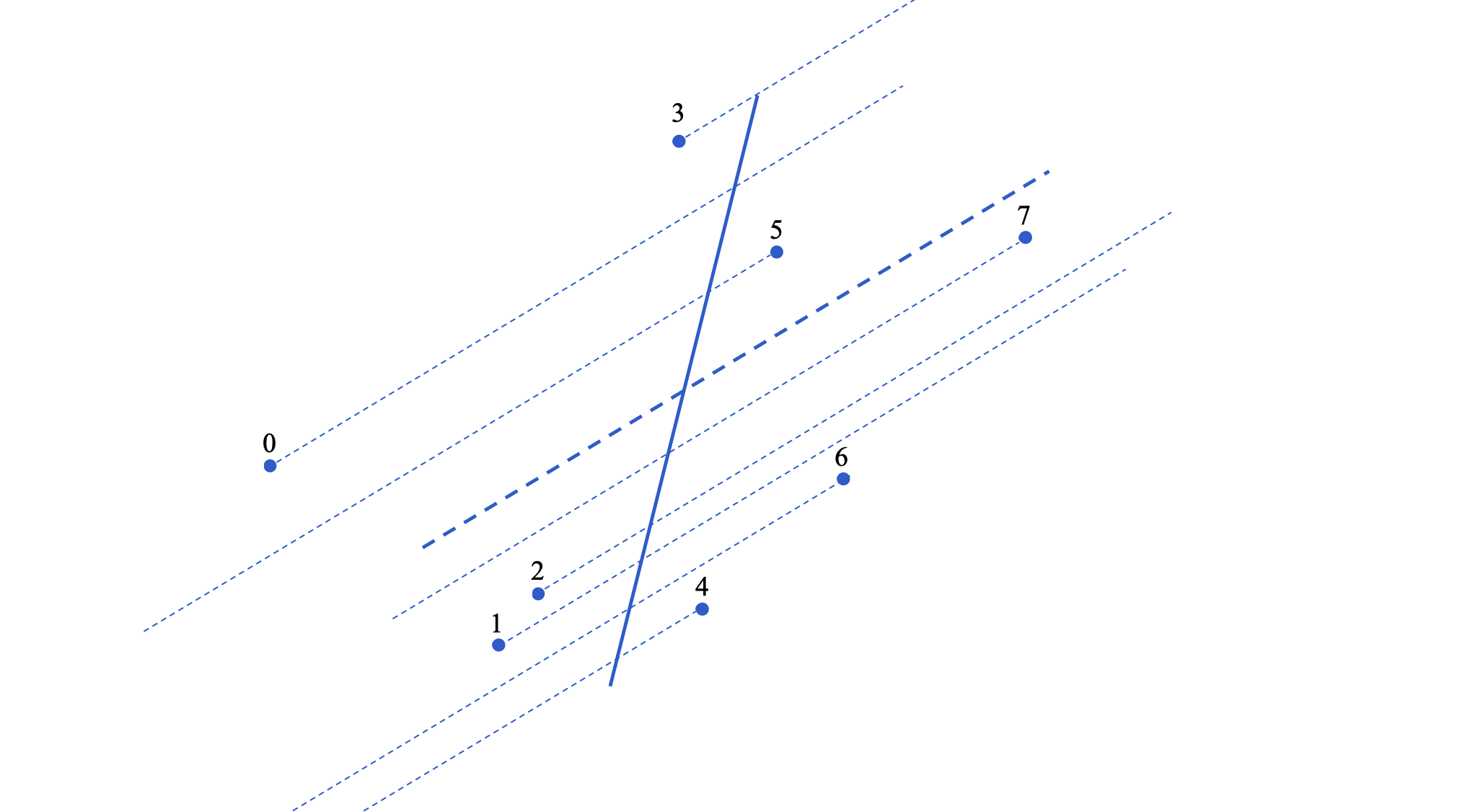
我们将投影点 按纵坐标从小到大排序,得到新坐标的索引,如上图中:
- 原坐标索引为 的点,新坐标索引为 ;
- 原坐标索引为 的点,新坐标索引为 ;
为了更直观地展示,将 来自左半部分的映射索引标记在直线左侧,将 来自右半部分的映射索引标记在直线右侧:
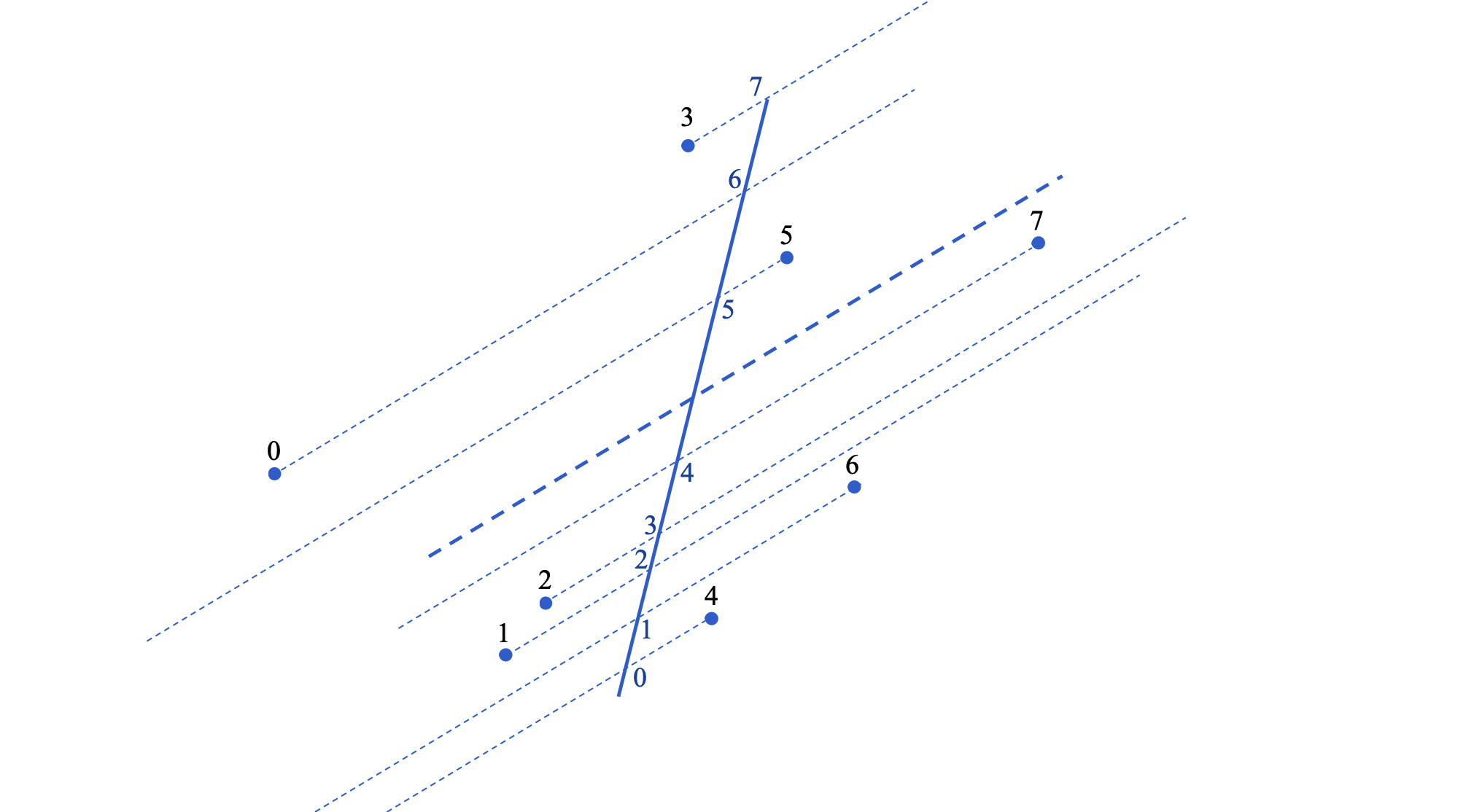
因为直线 已经将平面上的点平均分为左右两部分,因此 左侧和右侧肯定各有 个标记,想要找到 ,须有直线 的 左下、右下、左上、右上 各有 个标记:
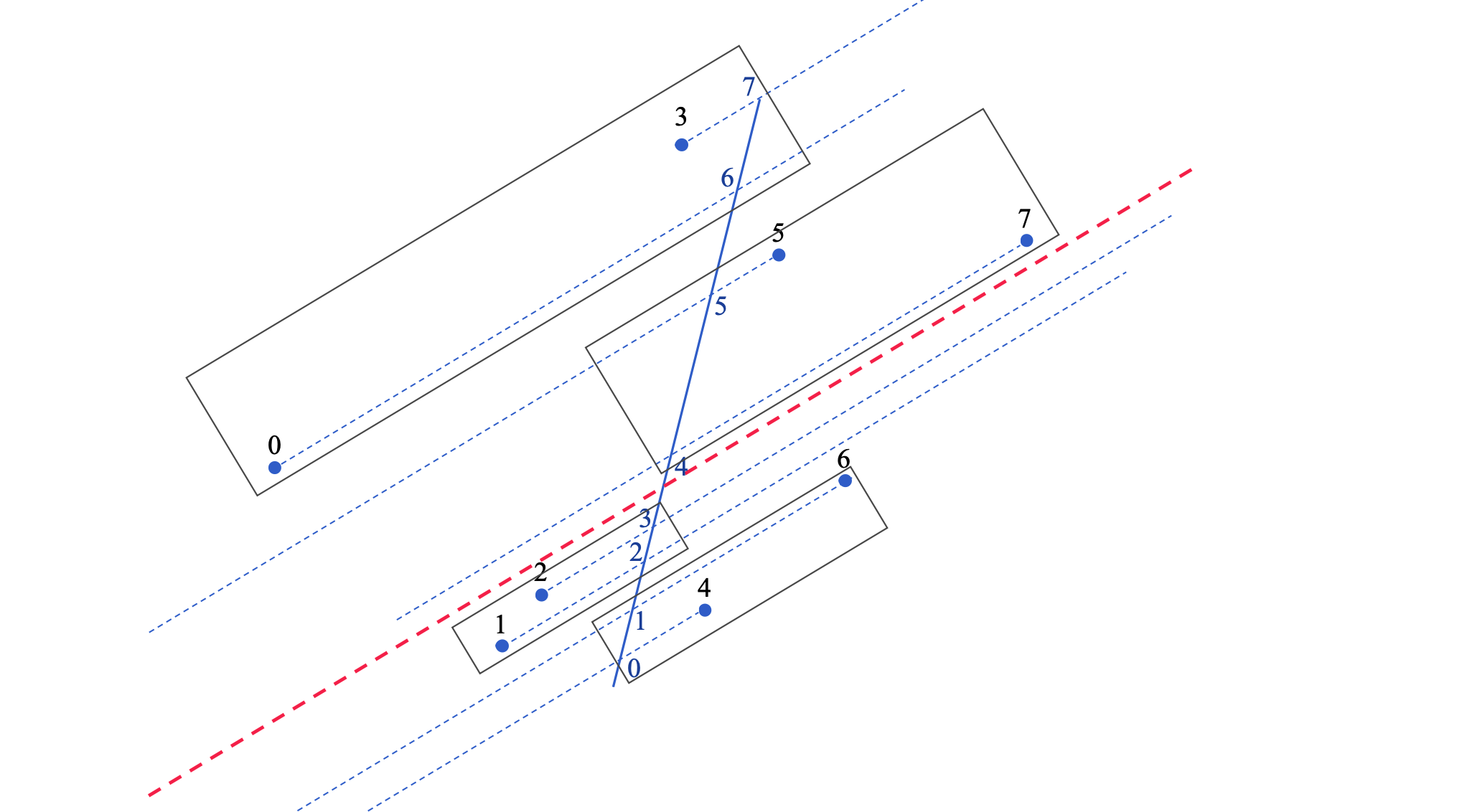
观察它们的原索引和新索引,我们可以发现:
- 在直线 左侧 = 原索引不超过
- 在直线 下方 = 新索引不超过
表现为代码,我们只需要按照 新坐标从小到大遍历,记录上述两种点位于左侧还是右侧,当位于左右两侧的点的个数同时达到 时,可以认为 存在。
如下图所示,当右侧的点率先超过 而左侧尚未达到时,说明当前斜率下 左侧下方缺点,因此我们考虑 左侧抬升以使得左下方容纳更多的点:
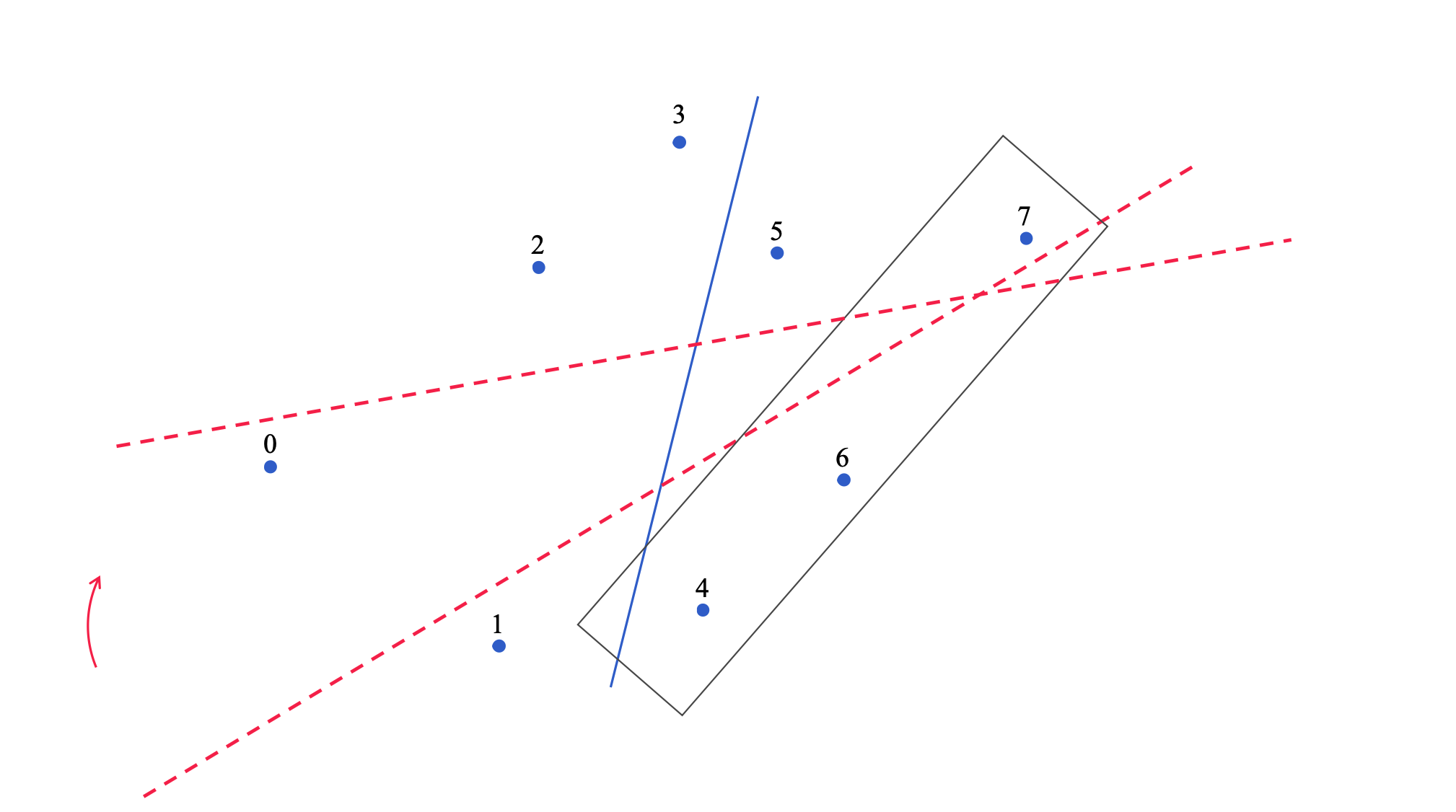
如何使用代码表达
原理讲清楚了,接下来就面临着 如何从数学上懂了转变为代码上懂了。
点的表达
我们采用结构体来表示点:
1 | struct Point{ |
此外,我们还需要另一种数据结构来同时存储点的新、旧索引:
1 | struct Ponit_with_i{ |
直线的表达
我们需要通过 两点确定一条直线 的方法来定义直线:
1 | struct Line{ |
此外,我们还需要确定 点根据指定斜率在直线上的投影,由于 点和斜率可以确定一条直线,进而将问题转化为 求两条直线的交点:
对于任意两条直线 和 ,通过解方程组使用参数表达 ,可得:
将其定义为一个函数:
1 | Point getCrossPoint(Line l1, Line l2){ // 计算两条直线的交点 |
double的精度控制
此外,由于本题涉及双浮点数的计算,我们习惯性地定义一个精度控制函数,来实现 等于 关系的比较:
1 | inline int dcmp(double x){ |
题解
1 |
|